Today we will learn how to customise standard cheque report and add a new cheque design.
1) Make a new Output type MenuItem for your cheque report.
2) Make changes in the standard enum for cheque report and add your cheque name.
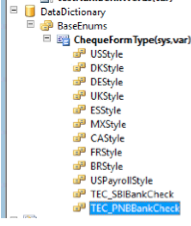
3) Make changes in chequeController Class. Add your report name in declaration section.
Call your report according to the Enum specified. The Enum will be the one you created above.
4) Make a method in Global class for converting numerals to Indian format
/// <summary>
/// Convert numerals to Hindi format text.
/// </summary
/// <param name="_num">
/// The numeral to be converted.
/// </param>
/// <returns>
/// The converted string.
/// </returns>
static TempStr TEC_numeralsToTxt_IN(real _num)
{
int numOfPennies = frac(_num)*100 mod 100;
int test = real2int(round(_num,0));
int paise;
int numOfTenths;
str 20 ones[19], tenths[9], hundreds, thousands, lakhs, crores, millions, billions;
str 40 textpaise;
int tmpnumofpennies;
int temp;
str 200 returntxt;
int checkPower(int _test, int _power)
{
int numOfPower;
if (_test >= _power)
{
numOfPower = _test DIV _power;
if (numOfPower >= 100)
{
temp = numOfPower DIV 100;
returntxt = returntxt + ' ' + ones[temp] + ' ' + hundreds;
numOfPower = numOfPower MOD 100;
}
if (numOfPower >= 20)
{
temp = numOfPower DIV 10;
returntxt = returntxt + ' ' + tenths[temp];
numOfPower = numOfPower MOD 10;
}
if (numOfPower >= 1)
{
returntxt = returntxt + ' ' + ones[numOfPower];
numOfPower = numOfPower MOD 10;
}
switch(_power)
{
case 1000000000 :
{
returntxt = returntxt + ' ' + billions;
_test = _test MOD 1000000000;
break;
}
case 10000000 :
{
returntxt = returntxt + ' ' + crores;
_test = _test MOD 10000000;
break;
}
case 100000 :
{
returntxt = returntxt + ' ' + lakhs;
_test = _test MOD 100000;
break;
}
case 1000 :
{
returntxt = returntxt + ' ' + thousands;
_test = _test MOD 1000;
break;
}
case 100 :
{
returntxt = returntxt + ' ' + hundreds;
_test = _test MOD 100;
break;
}
}
}
return _test;
}
ones[1] = "@SYS26620";
ones[2] = "@SYS26621";
ones[3] = "@SYS26622";
ones[4] = "@SYS26626";
ones[5] = "@SYS26627";
ones[6] = "@SYS26628";
ones[7] = "@SYS26629";
ones[8] = "@SYS26630";
ones[9] = "@SYS26631";
ones[10] = "@SYS26632";
ones[11] = "@SYS26633";
ones[12] = "@SYS26634";
ones[13] = "@SYS26635";
ones[14] = "@SYS26636";
ones[15] = "@SYS26637";
ones[16] = "@SYS26638";
ones[17] = "@SYS26639";
ones[18] = "@SYS26640";
ones[19] = "@SYS26641";
tenths[1] = 'Not used';
tenths[2] = "@SYS26643";
tenths[3] = "@SYS26644";
tenths[4] = "@SYS26645";
tenths[5] = "@SYS26646";
tenths[6] = "@SYS26647";
tenths[7] = "@SYS26648";
tenths[8] = "@SYS26649";
tenths[9] = "@SYS26650";
hundreds = "@SYS26651";
thousands = "@SYS26652";
lakhs = "Lakh";
crores = "Crore";
millions = "@SYS26653";
billions = "@SYS26654";
test = checkPower(test, 1000000000);
test = checkPower(test, 10000000);
test = checkPower(test, 100000);
test = checkPower(test, 1000);
test = checkPower(test, 100);
if (test >= 20)
{
numOfTenths = test DIV 10;
returntxt = returntxt + ' ' + tenths[numofTenths];
numOfTenths = numOfTenths MOD 10;
test = test MOD 10;
}
if (test >= 1)
{
numOfTenths = test;
returntxt = returntxt + ' ' + ones[numOfTenths];
}
if (numOfPennies)
{
returntxt = returntxt + ' ' + "@SYS5534" + "\n\n";
}
paise=numofPennies;
if(paise)
{
if(paise >=20)
{
numofTenths= paise DIV 10;
returntxt=returntxt + ' '+tenths[numofTenths];
numofTenths = numofTenths MOD 10;
paise=paise MOD 10;
}
if(paise >=1)
{
numOfTenths=paise;
returntxt=returntxt+ ' '+ones[numOfTenths];
}
returntxt=returntxt+" Paise";
}
return returntxt + " Only";
}
5) Next is CustVendCheque class. Initialize your Enum.
Here we converted the amount to Indian format.
6) Make Change in BankPrintTestCheque Class
7) Make Changes in BankChequePrint Class
8) Make a new report project in visual studio and add report and add dataset selecting ChequeDp class.
9) Add new design.
10) size should be the same as of your cheque. Here it is 8 X 4. Set accordingly.